Humanoid: Stair Climbing Motion
Stair climbing is different from normal walking motion because the robot has to move forward as well as vertically. Moving vertically is particularly challenging because the robot has to overcome the gravitational force acting downward on its COM while still maintaining balance. The motion described here is a statically stable motion which means the robot moves its joints slowly enough such that the dynamic forces due to joint motion can be neglected. So in order to balance the robot during the motion, it has to keep its center of mass within the foot support region. The size of stairs the robot can climb depends on the joint limitations.
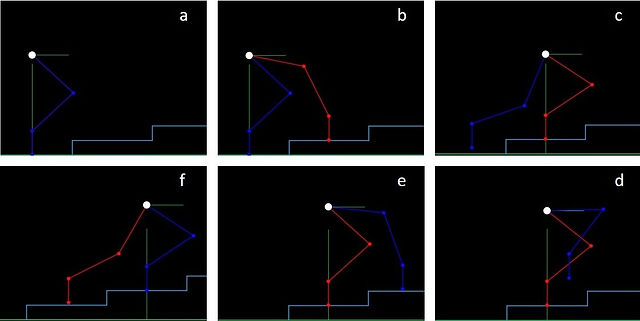
The robot legs are represented by blue (right leg) & red (left leg) bars. The waist is represented by white dot. The COM position is represented by vertical green line (a)The robot approaches the stairs & lowers waist height, (b)Robot places left foot on first stair, (c)Robot shifts COM from right foot to left foot, (d)Right leg in swing phase about to be placed on third stair, (e)Robot places right foot third stair with COM on left foot, (f)Robot shifts COM from left foot to right foot
The stair climbing gait is a combination of various postures. The robot is statically stable in all those postures. The entire sequence or postures can be broadly divided into two groups: (1) First moving the foot from one stair to another stair, (2) Shifting center of mass from one foot to another. The center of mass shifting takes place in 3D as it has to move both vertically as well as horizontally. The detailed sequence is described below:
-
Shift center of mass laterally to balance the robot on one leg i.e center of mass is now over a single foot
-
Move the other leg onto the first stair
-
Shift center of mass the other side laterally as well as longitudinally so that the center of mass is now over the newly placed foot in double support phase
-
Lift itself one stair height while balancing on one leg
-
Move the first leg onto second stair
-
Shift center of mass laterally as well as longitudinally on to next foot in double support phase
-
Repeat step 3 - 6 for each leg alternately
The COM lateral shifting is achieved through a sinusoidal function
Pseudo code for Stair climbing
Given below is the pseudo code for the robot climbing stairs of length 70 mm & height 20 mm. Let's go over what some of the variables & functions mean in the program.
-
(sx, sy) represent set of five points which form the trajectory of the foot when the robot places the first foot on one stair from rest position (figure sc 1 (b), for the red leg).
-
(s2x, s2y) represent set of five points which form the trajectory of the second foot (figure sc 1 (d), for the blue leg). This trajectory is repeated for climbing stairs.
-
"leg" keeps track of which leg is currently moving.
-
"rpos" represents the position of COM (waist) in the x-direction (direction of travel).
-
(xw, hw) represent position of COM (waist).
-
(xa_r, ha_r) & (xa_l, ha_l) represent position of ankle joints of right & left legs respectively.
-
sinetilt () function shifts COM laterally.
-
lagrange () function forms trajectory from points (sx, sy) or (s2x, s2y).
-
IK() is the inverse kinematic function which determines joint angles for the known position of foot.
Given below is the pseudo code for the robot climbing stairs of length 70 mm & height 20 mm. Let's go over what some of the variables & functions mean in the program.
//foot trajectory for first sequence
float sx[5]={0,25,50,60,70};
float sy[5]={55,100,90,82,75};
//foot trajectory for repeatable sequence
float s2x[5]={0,20,100,110,140};
float s2y[5]={55,90,110,105,75};
modify_pos(-30,0); //decrease the height by 30 mm to facilitate more foot travel
//initiate first step
for (int tilt=0;tilt<90;tilt++)
{
sinetilt(1.0,st_tilt,15); //shift COM sideways to balance on one foot
}
//take first step
for(int s_clmb=0;s_clmb<70;s_clmb++)
{
if (s_clmb<35)
{
xa_l=xa_l+2.0;
x=xa_l-rpos;
ha_l=gh-(lagrange(x,sx,sy));
}
else
{
xw=xw+2;
sinetilt(5.15,st_tilt,15);
}
//Inverse kinematics
IK(0); //inverse kinematics calculation for both legs
leg_motors_actuate(); //command motors to move to the required position
delay(50);
}
//execute repeatable step
for (int s_clmb=0;s_clmb<st_freq;s_clmb++)
{
for (int exe=0;exe<125;exe++)
{
if (leg==1)
{
if (exe<20)
{
hw=hw-1;
//inverse kinematics
IK(1); //inverse kinematics for right leg
}
else if(exe>=20 && exe<90)
{
xa_r=xa_r+2.0;
x=xa_r-rpos;
ha_r=gh-(lagrange(x,s2x,s2y))-ry;
//inverse kinematics
IK(0);
}
else
{
xw=xw+2;
//inverse kinematics
IK(0);
sinetilt(5.15,st_tilt,15);
}
leg_motors_actuate();
delay(50);
}
else
{
if (exe<20)
{
hw=hw-1;
//inverse kinematics
IK(-1); //inverse kinematics for left leg
}
else if(exe>=20 && exe<90)
{
xa_l=xa_l+2.0;
x=xa_l-rpos;
ha_l=gh-(lagrange(x,s2x,s2y))-ry;
//inverse kinematics
IK(0);
}
else
{
xw=xw+2;
//inverse kinematics
IK(0);
sinetilt(5.15,st_tilt,15);
}
leg_motors_actuate();
delay(50);
}
}
leg=leg*(-1);
ry+=20;
if (leg==-1)
{
rpos=xa_l;
}
else
{
rpos=xa_r;
}
}